Chapter 13: Area filling with GKS and Softfill
Previous chapter LLUs Home Next chapter Index
NCAR Graphics provides two methods for filling areas. The simplest is to use GKS calls directly to fill an area. However, the Softfill utility gives you much more flexibility and many more fill options than GKS.
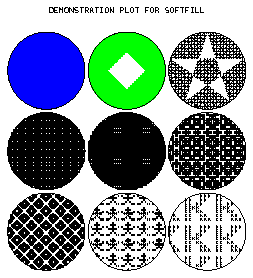
This module organizes the GKS area-filling routines and all Softfill routines according to their functions.
- GSFAIS
- Establishes the interior fill style to be used in subsequent calls to GFA.
- GFA
- Fills the specified polygon with the fill style set by the last call to GSFAIS.
- SFNORM
- Fills a polygon defined in NDCs with evenly spaced parallel lines or with rectangular patterns of dots or characters.
- SFWRLD
- Fills a polygon defined in world coordinates with evenly spaced parallel lines or with rectangular patterns of dots or characters.
- SFSGFA
- Fills a polygon defined in world coordinates with solid color fill (if available on your graphics device); otherwise it uses a suitable pattern fill.
- SFSETC
- Sets character parameters.
- SFSETI
- Sets integer parameters.
- SFSETR
- Sets real parameters.
- SFSETP
- Sets the dot pattern parameter.
- SFGETC
- Gets character parameters.
- SFGETI
- Gets integer parameters.
- SFGETR
- Gets real parameters.
- SFGETP
- Retrieves the dot pattern parameter.
For a more complete description of Softfill parameters, see the softfill_params man page or the Softfill programmer document.
-----------------------------------------------------------
Parameter Brief description Fortran type
-----------------------------------------------------------
AN ANgle of fill lines Real
CH CHaracter selector Integer or Character
DO DOt fill selector Integer
SP SPacing between fill lines Real
TY TYpe of fill selector Integer
LDP Dot pattern 8 x 8 Integer array
-----------------------------------------------------------
This module describes the two calls you must make to fill an area using GKS calls. GSFAIS sets the style or type of fill to be used, and GFA fills an area defined in world coordinates.
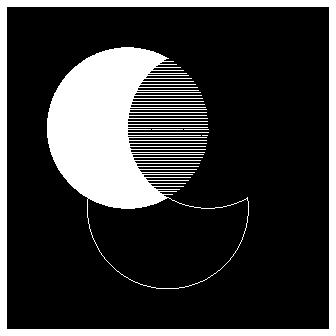
1 CALL GSFAIS (MOD (ICOLOR, 4))
2 CALL GFA (NC-1, XC, YC)
CALL GSFAIS (ISTYLE)
CALL GFA (N, X, Y)
- ISTYLE
- Integer, Input---The style of fill to be used in subsequent calls to GFA.
- 0
- Hollow fill. This is the default.
- 1
- Solid fill.
- 2
- Pattern fill: not implemented in NCAR Graphics GKS.
- 3
- Hatch fill.
- N
- Integer, Input---The number of points in the polygon to be filled. N must be greater than 2.
- X(N), Y(N)
- Real arrays, Input---The X and Y world coordinates of the polygon to be filled.
Notice that "hollow fill" is the default GKS fill style. If you don't call GSFAIS before calling GFA, as shown in the fcirc example, your polygons or areas will not be filled---in other words, they will be filled with nothing. This is the most common mistake that users make when trying to fill areas using GKS.
Line 1 of the fcirc.f code segment sets the fill style to be one of the four styles, and in line 2 we fill the areas. Hollow fill is used for the bottom portion of the lower circle, solid fill is used for the left portion of the left circle, pattern fill is used as the right portion of the right circle, and is empty because NCAR Graphics does not support this option, and hatch fill is used in the intersection of the two upper circles.
Softfill relies on parameters to control such things as fill patterns, distance between fill lines, and angles of crosshatching. There are eight routines to help you retrieve and set parameter values.
CALL SFSETC (PNAM, STRING)
CALL SFSETI (PNAM, IVAL)
CALL SFSETR (PNAM, RVAL)
CALL SFSETP (IARRAY)
CALL SFGETC (PNAM, STRING)
CALL SFGETI (PNAM, IVAL)
CALL SFGETR (PNAM, RVAL)
CALL SFGETP (IARRAY)
- SFSETC
- Gives a character value to a parameter.
- SFSETI
- Gives an integer value to a parameter.
- SFSETR
- Gives a real value to a parameter.
- SFSETP
- Sets the dot pattern array.
- SFGETC
- Retrieves character parameter information.
- SFGETI
- Retrieves integer parameter information.
- SFGETR
- Retrieves real parameter information.
- SFGETP
- Retrieves the dot pattern array.
- PNAM
- Character, Input---A character expression that represents a parameter name. Softfill uses only the first two characters in the string. Many users include additional characters that describe the parameter to make their code easier to maintain.
- IVAL
- Integer, Input or Output---An integer value or integer variable.
- RVAL
- Real, Input or Output---A real value or real variable.
- STRING
- Character expression, Input or Output---A character string or a character variable.
- IARRAY
- Integer array, Input or Output---An 8 x 8 integer array.
Note: To avoid a common user problem, be sure to double-check your "get" and "set" calls to ensure that you use the correct routine with the correct variable type. For example, do not use SFSETI to set a real parameter value.
SFNORM is used to fill polygons or areas with evenly spaced parallel lines, rectangular patterns of dots, or with rectangular patterns of single characters. SFNORM should be used when the points defining the areas to be filled are in NDCs.
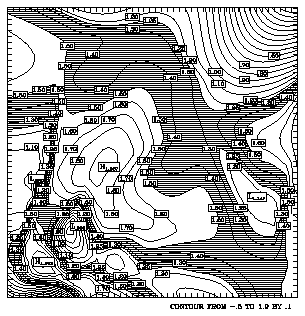
1 DO 10, I=1, NGRPS
2 IF (IGRP(I) .EQ. 3) IAREA3 = IAREA(I)
3 10 CONTINUE
4 IF (IAREA3 .EQ. 1) THEN
5 CALL SFSETR ('SP - SPACING BETWEEN FILL LINES', .006)
6 CALL SFNORM (XWRK, YWRK, NWRK, RSCR, 5000, ISCR, 5000)
7 ENDIF
CALL SFNORM (XWRK, YWRK, NWRK, DST, NST, IND, NND)
- XWRK(NWRK), YWRK(NWRK)
- Real arrays, Input---The X and Y coordinates of the points that define the boundary of the polygon to be filled. Values in these arrays must be in NDCs. You do not need to repeat point 1 at the end of the boundary to close the polygon.
- NWRK
- Integer, Input---The number of points defining the area to be filled. NWRK must have a value greater than 2.
- DST(NST)
- Real array, Workspace.
- NST
- Integer, Input---The length of the real workspace array. Make NST>=NWRK+NIM, where NWRK is the number of points defining the area to be filled, and NIM is the largest number of intersection points of any fill line with the boundary lines. To be sure DST is dimensioned large enough, you can use NIM=NWRK; in practice, NIM rarely needs to be that large.
- IND(NND)
- Integer array, Workspace.
- NND
- Integer, Input---The length of the integer workspace array. Make NND>=NWRK+2*NIM, where NWRK is the number of points defining the area to be filled, and NIM is the number of intersection points of any fill line with the boundary lines. To be sure IND is dimensioned large enough, you can use NIM=NWRK; in practice, NIM rarely needs to be that large.
In practice, SFNORM is most frequently called by a user-supplied subroutine called by the Areas routine ARSCAM. Because ARSCAM passes the fill routine polygon coordinates in NDCs, SFNORM should be used to do pattern fill. The Areas and Conpack chapters of the NCAR Graphics Contouring and Mapping Tutorial provide several examples of how to write this fill routine; see module "Ar 3.5 Writing an area-fill routine" for one example.
The code segment for the ccpscam example is from the routine SFILL, which is a user-supplied subroutine called by the Areas routine ARSCAM to fill certain contour levels. Lines 1 through 3 of the ccpscam.f code segment retrieve the area identifier for the contour level. Line 4 checks to see if the area identifier is 1, as we set the area identifier for the shaded area to be. If it is, then line 5 sets the Softfill spacing option, and line 6 calls SFNORM to fill the contour level.
Note: SFNORM does not draw the boundaries around an area. To draw the boundaries, use CURVED or the appropriate line-drawing routine for the utility. In the ccpscam example, CPCLDR is used to draw contour lines.
SFWRLD is used to fill polygons or areas with evenly spaced parallel lines, rectangular patterns of dots, or rectangular patterns of single characters. SFWRLD should be used when the points defining the areas to be filled are in world coordinates.
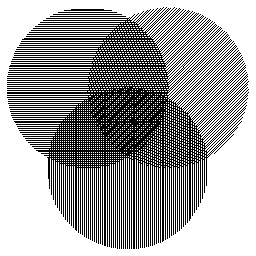
1 DO 100, II=1, NPTS
2 ANG = D2R * 3.6 * FLOAT (II-1)
3 X = COS (ANG)
4 Y = SIN (ANG)
5 X1(II) = X - .5
6 Y1(II) = Y + .5
7 100 CONTINUE
8 CALL SFSETR ('SP - SPACING BETWEEN FILL LINES', 0.006)
9 CALL SFSETR ('AN - ANGLE OF FILL LINES', 0.)
10 CALL SFWRLD (X1, Y1, NPTS, RWRK, LRWK, IWRK, LIWK)
11 CALL SFSETR ('AN - ANGLE OF FILL LINES', 45.)
12 CALL SFWRLD (X2, Y2, NPTS, RWRK, LRWK, IWRK, LIWK)
13 CALL SFSETR ('AN - ANGLE OF FILL LINES', 90.)
14 CALL SFWRLD (X3, Y3, NPTS, RWRK, LRWK, IWRK, LIWK)
CALL SFWRLD (XWRK, YWRK, NWRK, DST, NST, IND, NND)
- XWRK(NWRK), YWRK(NWRK)
- Real arrays, Input---The X and Y world coordinates of the points that define the boundary of the polygon to be filled. Values in these arrays must be in world coordinates. You do not need to repeat point 1 at the end of the boundary to close the polygon.
- NWRK
- Integer, Input---The number of points defining the area to be filled. NWRK must have a value greater than 2.
- DST(NST)
- Real array, Workspace.
- NST
- Integer, Input---The length of the real workspace array. Make NST>=NWRK+NIM, where NWRK is the number of points defining the area to be filled, and NIM is the largest number of intersection points of any fill line with the boundary lines. To be sure DST is dimensioned large enough, you can use NIM=NWRK; in practice, NIM rarely needs to be that large.
- IND(NND)
- Integer array, Workspace.
- NND
- Integer, Input---The length of the integer workspace array. Make NND>=NWRK+2*NIM, where NWRK is the number of points defining the area to be filled, and NIM is the number of intersection points of any fill line with the boundary lines. To be sure IND is dimensioned large enough, you can use NIM=NWRK; in practice NIM rarely needs to be that large.
In practice, SFWRLD is most frequently called directly to shade a region for which the user already knows the boundary points. Lines 1 through 7 of the fsfwrld.f code segment establish points that define the circle containing horizontal fill lines. Line 10 fills this circle with horizontal lines. Lines 8 and 9 set parameters to control fill line spacing and the angle from horizontal used for drawing the fill lines. Lines 11 through 14 fill two other circles with lines at different angles.
Notice that the boundaries around the circles are not drawn. Neither SFWRLD nor SFNORM will draw the boundary lines around an area. If you need boundary lines, draw them using CURVED, or with an appropriate line-drawing routine for the specific utility you are currently using (such as CPCLDR to draw contour lines in Conpack).
SFSGFA provides a way to use hardware-controlled solid color fill (if that capability is available on your graphics device); otherwise it uses a suitable software-controlled pattern fill. Using SFSGFA gives you the advantage of being able to change how areas are filled by modifying only a single variable.
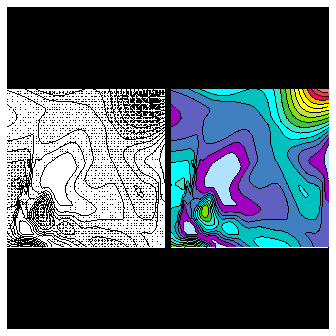
1 SUBROUTINE SFILL (XWRK, YWRK, NWRK, IAREA, IGRP, NGRPS)
2
3 REAL XWRK(*), YWRK(*), ISCR(5000)
4 INTEGER IAREA(*), IGRP(*), RSCR(5000)
5
6 DO 10, I=1, NGRPS
7 IF (IGRP(I) .EQ. 3) IAREA3 = IAREA(I)
8 10 CONTINUE
9 IF (IAREA3 .GE. 1) THEN
10 CALL SFSGFA (XWRK, YWRK, NWRK, RSCR, 5000, ISCR, 5000, IAREA3 + 1)
11 ENDIF
CALL SFSGFA (XRA, YRA, NRA, DST, NST, IND, NND, ICI)
- XRA(NRA), YRA(NRA)
- Real arrays, Input and Output---Contain the X and Y coordinates of the points that define the boundary of the polygon to be filled. Use the world coordinate system for input values. After a call to SFSGFA, this array contains the X and Y coordinates transformed to NDCs. You do not need to repeat point 1 at the end of the boundary to close the polygon.
- NRA
- Integer, Input---The number of points defining the area to be filled. NRA must have a value greater than 2.
- DST(NST)
- Real array, Workspace---A real scratch array, dimensioned NST. This array is used only when SFSGFA makes calls to SFWRLD and/or SFNORM to generate fill lines.
- NST
- Integer, Input---The dimension of DST. Make NST>=NRA+NIM, where NRA is the number of points defining the area to be filled, and NIM is the largest number of intersection points of any fill line with the boundary lines. To be sure DST is dimensioned large enough, you can use NIM=NRA; in practice, NIM rarely needs to be that large. For example, NIM=2 is sufficient for an octagon.
- IND(NND)
- Integer array, Workspace---This integer scratch array is used only when SFSGFA makes calls to SFWRLD and/or SFNORM to generate fill lines.
- NND
- Integer, Input---The dimension of IND. Make NND>=NRA+2*NIM, where NRA is the number of points defining the area to be filled, and NIM is the number of intersection points of any fill line with the boundary lines. To be sure IND is dimensioned large enough, you can use NIM=NRA; in practice, NIM rarely needs to be that large.
- ICI
- Integer, Input---ICI is a fill area index used to determine how an area is filled. The value of the internal parameter TY directly affects the function of ICI. See the next module for more information about TY.
- TY=0
- SFSGFA does color fill by calling GKS routine GFA. This is the default value.
- ICI>=0 specifies the color index of the fill area.
- ICI<0 specifies that the fill area color index is not to be set before calling GFA.
- TY=1
- SFSGFA fills the area with parallel lines spaced SP units apart, and at the angle AN, by calling SFWRLD.
- ICI>=0 specifies the polyline color index.
- ICI<0 specifies that the polyline color index is not to be set before calling SFWRLD.
- Note: If CH and DO are set to select dot fill or character fill, the values of ICI will not affect the color of the dots or characters.
- TY=2
- SFSGFA calls SFWRLD to fill the area with parallel lines at the angle AN, and calls SFNORM to fill the area again with parallel lines at the angle AN+90; all lines are spaced SP units apart.
- ICI>=0 specifies the polyline color index.
- ICI<0 specifies that the polyline color index is not to be set before calling SFWRLD.
- Note: If CH and DO are set to select dot fill or character fill, the values of ICI will not affect the color of the dots or characters.
- TY = -4, -3, -2, -1
- SFSGFA fills the area with line patterns by calling SFWRLD and/or SFNORM. The ABS(TY) determines the maximum number of fill-line angles used in a pattern. ICI determines the density of the lines drawn at each angle.
In most cases, it is relatively easy to use SFSGFA. Since the Areas routine ARSCAM sets world and NDC coordinates to be the same before calling the processing or fill routine, SFSGFA can be used in place of SFNORM for most Areas applications. The argument list for SFSGFA is identical to those of SFNORM and SFWRLD, except that it has an extra argument, ICI. It is easiest to think of ICI as a fill index, which does the appropriate thing if the parameter TY is set.
In the fsfsgfa example, SFILL is called from the driver program by Areas routine ARSCAM. Line 10 of the fsfsgfa.f code segment calls SFGSFA to fill each area. The argument ICI in SFGSFA is set equal to the area identifier in SFILL, so that the fill option is related to the area being filled. In the next module, we examine the driver program to see how to get both black and white cross-hatching and color fill with the same call to SFGSFA.
When TY<>0, ICI is used to select the density of lines in each direction. A zero or negative value of ICI selects a blank pattern. Positive values of ICI select patterns that increase in density as ICI increases from 0 to 15. The largest usable value of ICI is approximately 5*ABS(TY); beyond that, the pattern becomes essentially solid. For example, if TY=-4, then 20 is about the largest value of ICI that you can use and still see a pattern.
For each increase in ICI, fill lines are added at one of the usable angles. The first time lines are added at a given angle, they are added between existing lines so that the distance between lines at that angle is halved. An ICI value that is evenly divisible by the absolute value of TY yields a pattern that is evenly dense at all angles. For example, if TY=-2, the patterns associated with the first three values of ICI are formed as follows: ICI=1 uses lines at the angle AN, spaced 32*SP units apart; ICI=2 uses lines at the angles AN and AN+90, both spaced 32*SP units apart; ICI=3 uses lines at AN, spaced 32*SP units apart, and lines at AN+90, spaced 16*SP units apart.
The parameter TY controls exactly what type of fill you get when you call a Softfill routine.
1 CALL CPRECT (ZREG, MREG, MREG, NREG, RWRK, LRWK, IWRK, LIWK)
2 CALL SFSETI ('TY - TYPE OF FILL', -4)
3 CALL CPBACK (ZREG, RWRK, IWRK)
4 CALL ARINAM (MAP, LMAP)
5 CALL CPCLAM (ZREG, RWRK, IWRK, MAP)
6 CALL ARSCAM (MAP, XWRK, YWRK, NWRK, IAREA, IGRP, NOGRPS, SFILL)
7 CALL CPCLDR (ZREG, RWRK, IWRK)
10 CALL CPRECT (ZREG, MREG, MREG, NREG, RWRK, LRWK, IWRK, LIWK)
11 CALL GSFAIS (1)
12 CALL SFSETI ('TY - TYPE OF FILL', 0)
13 CALL CPBACK (ZREG, RWRK, IWRK)
14 CALL ARINAM (MAP, LMAP)
15 CALL CPCLAM (ZREG, RWRK, IWRK, MAP)
16 CALL ARSCAM (MAP, XWRK, YWRK, NWRK, IAREA, IGRP, NOGRPS, SFILL)
17 CALL CPCLDR (ZREG, RWRK, IWRK)
CALL SFSETI ('TY', ity)
- TY
- Integer---The type of fill to be done by the routine SFSGFA.
- 0
- SFSGFA does color fill by calling GKS routine GFA. This is the default value.
- ICI>=0 specifies the color index of the fill area.
- ICI<0 specifies that the fill area color index is not to be set before calling GFA.
- 1
- SFSGFA fills the area with parallel lines spaced SP units apart, and at the angle AN, by calling SFWRLD.
- ICI>=0 specifies the polyline color index.
- ICI<0 specifies that the polyline color index is not to be set before calling SFWRLD.
- Note: If CH and DO are set to select dot fill or character fill, the values of ICI will not affect the color of the dots or characters.
- 2
- SFSGFA calls SFWRLD to fill the area with parallel lines and calls SFNORM to fill the area again with parallel lines perpendicular to the first set.
- ICI>=0 specifies the polyline color index.
- ICI<0 specifies that the polyline color index is not to be set before calling SFWRLD.
- Parameters AN, CH, DO, and SP can be used to further affect the nature of the fill. If CH and DO are set to select dot fill or character fill, the values of ICI will not affect the color of the dots or characters.
- -4, -3, -2, -1
- SFSGFA fills the area with line patterns by calling SFWRLD and/or SFNORM. The ABS(TY) determines the maximum number of fill-line angles used in a pattern. ICI determines the density of the lines drawn at each angle.
Lines 1 through 7 in the fsfsgfa.f code segment set up the area map and call Conpack to draw the contours. Line 2 chooses cross-hatching at four different angles as the type of fill for each contour level by setting TY=4. The lines are then drawn by SFGSFA in subroutine SFILL by the call to ARSCAM in line 6. The call to SFGSFA and the subroutine SFILL are discussed in the preceding module.
Lines 10 through 17 do exactly the same thing, however, this time, line 11 sets the GKS solid fill option, and line 12 sets solid color as the type of fill with TY=0. Line 16 fills the contour by calling ARSCAM, which in turn calls SFILL to do the filling. Notice that to change the type of fill, it is only necessary to change the value of the parameter TY.
There are two parameters to control fill lines: AN controls the angles at which lines are drawn, and SP controls the spacing between lines.
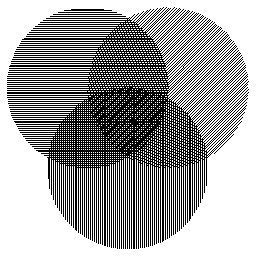
1 DO 100, II = 1, NPTS
2 ANG = D2R * 3.6 * FLOAT (II - 1)
3 X = COS (ANG)
4 Y = SIN (ANG)
5 X1(II) = X - .5
6 Y1(II) = Y + .5
7 100 CONTINUE
8 CALL SFSETR ('SP - SPACING BETWEEN FILL LINES', 0.006)
9 CALL SFSETR ('AN - ANGLE OF FILL LINES', 0.)
10 CALL SFWRLD (X1, Y1, NPTS, RWRK, LRWK, IWRK, LIWK)
11 CALL SFSETR ('AN - ANGLE OF FILL LINES', 45.)
12 CALL SFWRLD (X2, Y2, NPTS, RWRK, LRWK, IWRK, LIWK)
13 CALL SFSETR ('AN - ANGLE OF FILL LINES', 90.)
14 CALL SFWRLD (X3, Y3, NPTS, RWRK, LRWK, IWRK, LIWK)
CALL SFSETR ('AN', angle)
CALL SFSETR ('SP', space)
- AN
- Real---The angle, in degrees, at which fill lines are to be drawn. By default, AN=0.
- SP
- Real---The spacing between each fill line, or if dot fill is selected, the distance between any pair of adjacent dots along a fill line. The value is given in NDCs. For this reason, 0.0<=SP<=1.0. By default, SP=0.00125. This produces nearly solid fill on many devices.
Lines 1 through 7 of the fsfwrld.f code segment define the perimeters of the circles. Line 8 sets the spacing between fill lines to a reasonably large value, then line 9 specifies that the lines be drawn horizontally. Line 10 fills the first circle. Then using the same spacing, lines 11 and 12 fill the second circle with lines drawn at a 45 degree angle. Lines 13 and 14 fill the third circle with vertical fill lines.
Softfill uses two parameters and a pattern array to control two things: how areas are filled (dots, characters, or lines) and the pattern of the fill.
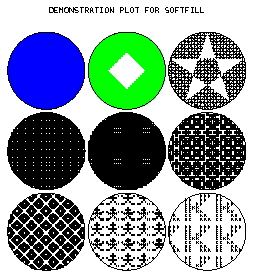
1 DATA ID4 / 0, 0, 0, 0, 0, 0, 0, 0,
2 + 0, 1, 1, 0, 0, 1, 1, 1,
3 + 0, 1, 1, 0, 0, 1, 1, 0,
4 + 0, 1, 1, 0, 1, 1, 0, 0,
5 + 0, 1, 1, 1, 1, 0, 0, 0,
6 + 0, 1, 1, 0, 1, 1, 0, 0,
7 + 0, 1, 1, 0, 0, 1, 1, 0,
8 + 0, 1, 1, 0, 0, 1, 1, 1/
9 CALL SFSETI ('DO - DOT-FILL FLAG', 1)
10 109 CALL GSCHH (.008)
11 CALL SFSETR ('SP - SPACING OF FILL LINES', .012)
12 CALL SFSETC ('CH - CHARACTER SPECIFIER', 'K')
13 CALL SFSETP (ID4)
14 CALL SFWRLD (XRA, YRA, 100, DST, 102, IND, 104)
CALL SFSETI ('DO', ido)
CALL SFSETC ('CH', char)
CALL SFSETP (IARRAY)
- DO
- Integer---The dot fill parameter determines whether dots, characters or lines are drawn.
- 0
- Solid lines are drawn. This is the default.
- 1
- Dots or characters are drawn.
- CH
- Integer or Character---The character parameter determines what is drawn at each dot.
- 0
- The fill character is a dot. This is the default.
- n>0
- The fill character is specified by CHAR(n), where n is the integer you specify.
- n<0
- The fill character is a GKS polymarker of type ABS(n), where n is the integer you specify:
-
-1 (dot)
-2 + (plus)
-3 * (asterisk)
-4 o (circle)
-5 x (cross)
- [a_character]
- The fill character is the ASCII character that you specify.
- IARRAY
- Integer array---An 8x8 array in which the dot pattern is to be set or retrieved. Each dot is associated with a particular element of IARRAY. If that element is a 1, then the dot is drawn; if it is a 0, then the dot is not drawn. The association is done so that the pattern is replicated across the entire filled area. By default, IARRAY consists of all 1s.
When dot fill is selected, each fill line is drawn using dots; if CH is nonzero, then each fill line is drawn using the specified character or symbol. Dots along a fill line are the same distance apart as the lines are from each other; these dots appear at the intersection points of a square grid.
If AN=0, then incrementing the first subscript of IARRAY corresponds to horizontal motion across the plot from left to right, and incrementing the second subscript of IARRAY corresponds to a vertical motion across the plot from top to bottom. This allows the contents of IARRAY to be declared in a DATA statement that creates a picture of the pattern, as shown in lines 1 through 8 of the tsoftf.f code segment.
By changing AN, it is possible to rotate the fill pattern. This dot pattern is used until another call to SFSETP changes the contents of the array. By setting CH, you can change the elements used to define the dot pattern.
Line 10 sets the character size, and line 12 sets the character to "K," so that small printed Ks define a K pattern in the lower right circle in the plot. Line 13 sets the K pattern with a call to SFSETP, and line 14 draws the pattern.
Previous chapter LLUs Home Next chapter Index