NCL commands
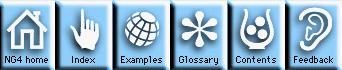

This module discusses those statements that are legal to type in--in
interactive mode--at the NCL prompt, or those statements that are legal
to include in an NCL script. This
module also details the functions and procedures that
are a part of NCL and how to add to that function set.
A statement is the fundamental element of NCL. Statements are not
restricted to being a single line of source code, and statements can be nested
within statements. You should keep two important things in mind about
how NCL executes its statements:
-
A statement is only executed after it has been parsed and is found
to be free of syntax errors.
-
If a runtime error occurs while executing a statement, execution of the
statment--and any statements in which the erroneous statement is
nested--is immediately terminated.
NCL offers several flow control statements that are similar to
flow control statements in other computer languages
(details are provided by the links):
-
If statements
- These statements, of the form
If-then and If-then-else, implement a decision-making
capability in NCL.
-
Looping - The two looping statements are
Do
for looping from a starting value to an ending value with a
stride and
Do while
for looping until a condition is met.
- break can
be used to abort a loop and
continue can
be used to abort the current iteration of a loop.
NCL has three statements for interfacing with the
High Level Utilities library:
-
create - Allows you to create an
HLU object and assign values to its resources.
-
setvalues - Allows you to modify resources in an
HLU object after it has been created.
-
getvalues - Allows you to retrieve the values of
the resource setting in an HLU object.
-
block allows you to group statements into a new statement
and can be used to require syntax-checking on a block of statements
before executing those statements.
- An
assignment statement allows you to assign a value
to any legal NCL construct
that can have a value, such as variables, arrays, and so forth.
- new
is a statement that can be used to initialize variables and arrays
and is similar in function to type statements in other languages.
- quit
is used to exit NCL.
-
record and
stop
can be used to start and stop the recording of NCL commands in a file.
In NCL, a function or procedure is a name followed by arguments in
parentheses with the arguments separated by commas. A function or
procedure is invoked by referencing its name and supplying an
argument list. A function returns a value, a procedure does not.
Arguments can be of any type, but facilities
exist in defining a function
for imposing restrictions on the arguments, such as numbers of
dimensions and dimension sizes. For arguments that must be a certain
type, the NCL
coercion rules apply. All arguments are passed by
reference in NCL; this means that a change in the value of an argument
within a function or procedure will change the value of the argument in
the call. If a parameter is coerced before a function is called,
changes within the function will not be reflected in the parameter.
- addfile
- Function used to open a new data file (returns a file id).
- all
- Function that returns True if all of the arguments in the input
logical array are True.
- any
- Function that returns True if any of the arguments in the
input logical array is True.
- asciiread
- Function that reads ASCII files.
- asciiwrite
- Procedure that writes an ASCII data file from an NCL numeric data type.
- cbinread
- Function that reads binary files created with a C block I/O function write.
- cbinwrite
- Procedure that writes a binary file in raw C block I/O format from a
numeric data type.
- delete
- Procedure used to delete variables and free the symbol name from
the symbol table.
- dimsizes
- Function that returns the sizes of the dimensions of any data array.
- fbinread
- Function that reads binary files created using an unformatted Fortran
write.
- fbinwrite
- Procedure that writes a binary file, using Fortran unformatted writes,
of a numeric data type.
- getenv
- Function that retrieves the values of shell environment variables.
- getfilevarnames
- Function that retrieves the variable names from a file.
- isatt
- Function that returns True if a specific attribute name is defined.
- isdim
- Function that returns True if a specific dimension name is defined.
- isfilevar
- Function that returns True if a specific file variable name is defined.
- isfilevaratt
- Function that returns True if a specific file variable attribute name is defined.
- isfilevardim
- Function that returns True if a specific file variable dimension name is defined.
- ismissing
- Function that returns True if its argument references a missing value.
- list_files
- Procedure that lists all of the current variables containing
references to files.
- list_filevars
- Procedure that lists all of the variables associated with a
specific file.
- list_hlus
- Procedure that lists all of the HLU objects currently referenced by NCL.
- list_procfuncs
- Procedure that lists all the currently defined NCL functions and
procedures.
- list_vars
- Procedure that lists the currently defined variables.
- print
- Procedure that prints the value of a variable (including file variables)
and expressions.
- qsort
- Procedure that sorts singly-dimensioned numeric arrays in place.
- sqsort
- Procedure that sorts singly-dimensioned string arrays in place.
- rand
- Function that generates pseudo random numbers.
- sizeof
- Function that returns the size, in bytes, of its argument.
- sleep
- Procedure that pauses execution for a specified number of seconds.
- srand
- Procedure that establishes a seed for the rand function.
- system
- Procedure that passes UNIX system commands to the shell.
Currently supported math functions are:
abs ,
acos ,
asin ,
atan ,
ceil ,
cos ,
cosh ,
fabs ,
floor ,
idsfft ,
log ,
log10 ,
sin ,
sinh ,
sqrt ,
tan ,
tanh
.
NCL contains versions of most of the functions and procedures available to the
HLUs. For some of these, such as "draw", "delete" and so forth, the NCL
names have been shortened for convenience. The full HLU names are still
available in NCL if you want to use them. You may use whichever name you
prefer; the arguments will be identical.
- clear
- Procedure that provides access to the NhlClearWorkstation procedure for
clearing workstations.
- datatondc
- Procedure that provides access to the function NhlDataToNDC for
converting from data coordinates to NDC coordinates.
- destroy
- Procedure that provides access to the NhlDestroy procedure for
removing HLU objects.
- draw
- Procedure providing access to the basic draw function NhlDraw.
- frame
- Procedure providing access to the HLU picture control function NhlFrame.
- ndctodata
- Procedure providing access to the function NhlNDCToData for converting
from NDC coordinates to data coordinates.
- NhlAddAnnotation
- Function that adds an annotation to a plot.
- NhlAddData
- Function for adding one or more additional data items to a plot.
- NhlClassName
- Function returning the class name of one or more HLU objects.
- NhlDataPolyline
- Procedure for drawing an immediate mode polyline using data coordinates.
- NhlDataPolygon
- Procedure for drawing an immediate mode polygon using data coordinates.
- NhlDataPolymarker
- Procedure for drawing an immediate mode polymarker using data coordinates.
- NhlFreeColor
- Procedure for removing color index settings from specified workstations.
- NhlGetBB
- Function for retrieving the bounding boxes of a list of HLU input
objects.
- NhlIsAllocatedColor
- Function for interrogating a workstation to see if a given color index
has been allocated.
- NhlName
- Function that returns the object name of an HLU object, given the HLU
object identifier.
- NhlNDCPolyline
- Procedure for drawing an immediate mode polyline using NDC coordinates.
- NhlNDCPolygon
- Procedure for drawing an immediate mode polygon using NDC coordinates.
- NhlNDCPolymarker
- Procedure for drawing an immediate mode polymarker using NDC coordinates.
- NhlNewColor
- Function used to allocate new workstation color indices.
- NhlRemoveAnnotation
- Procedure for removing annotations from plots.
- NhlRemoveData
- Procedure for removing data items from one or more plots.
- NhlRemoveOverlay
- Procedure for removing one or more plots from an overlay.
- NhlSetColor
- Procedure for defining workstation color indices.
- NhlUpdateData
- Procedure that forces an update of the internal state of a
DataComm object.
- overlay
- Procedure used to overlay one HLU plot object over a specific style of
transformation.
- update
- Procedure providing access to the HLU update workstation function
NhlUpdateWorkstation.
Conversion functions exist for every possible data conversion in
NCL:
chartodouble,
chartofloat,
chartoint,
chartolong,
chartoshort,
doubletobyte,
doubletochar,
doubletoint,
doubletofloat,
doubletolong,
doubletoshort,
floattobyte,
floattochar,
floattoint,
floattolong,
floattoshort,
inttobyte,
inttochar,
inttoshort,
longtobyte,
longtochar,
longtoint,
longtoshort,
shorttobyte,
shorttochar,
chartostring,
stringtochar.
An intrinsic function is not defined by NCL source; it is a C or
Fortran routine that has been added to the NCL function set.
Intrinsic functions often perform operations that NCL source does not support.
For details on NCL intrinsic functions, see the
Intrinsic functions section of the NCL Reference Guide.
NCL allows you to define your own functions and procedures.
NCL functions and procedures are similar to functions and procedures in most
programming languages, but there are some distinct differences. For details
on procedures in general, see
NCL procedure overview. For specifics on the syntax of defining your
own functions and procedures, see
NCL functions and procedures definitions.
Please see the NCL Reference Manual for a discussion of
Extending NCL's function set.
User Guide Control Panel
NG4.1 Home, Index, Examples, Glossary, Feedback, UG Contents, UG WhereAmI?
$Revision: 1.14 $ $Date: 1998/06/15 22:08:40 $